How to Create a Gradient Text Effect Using CSS
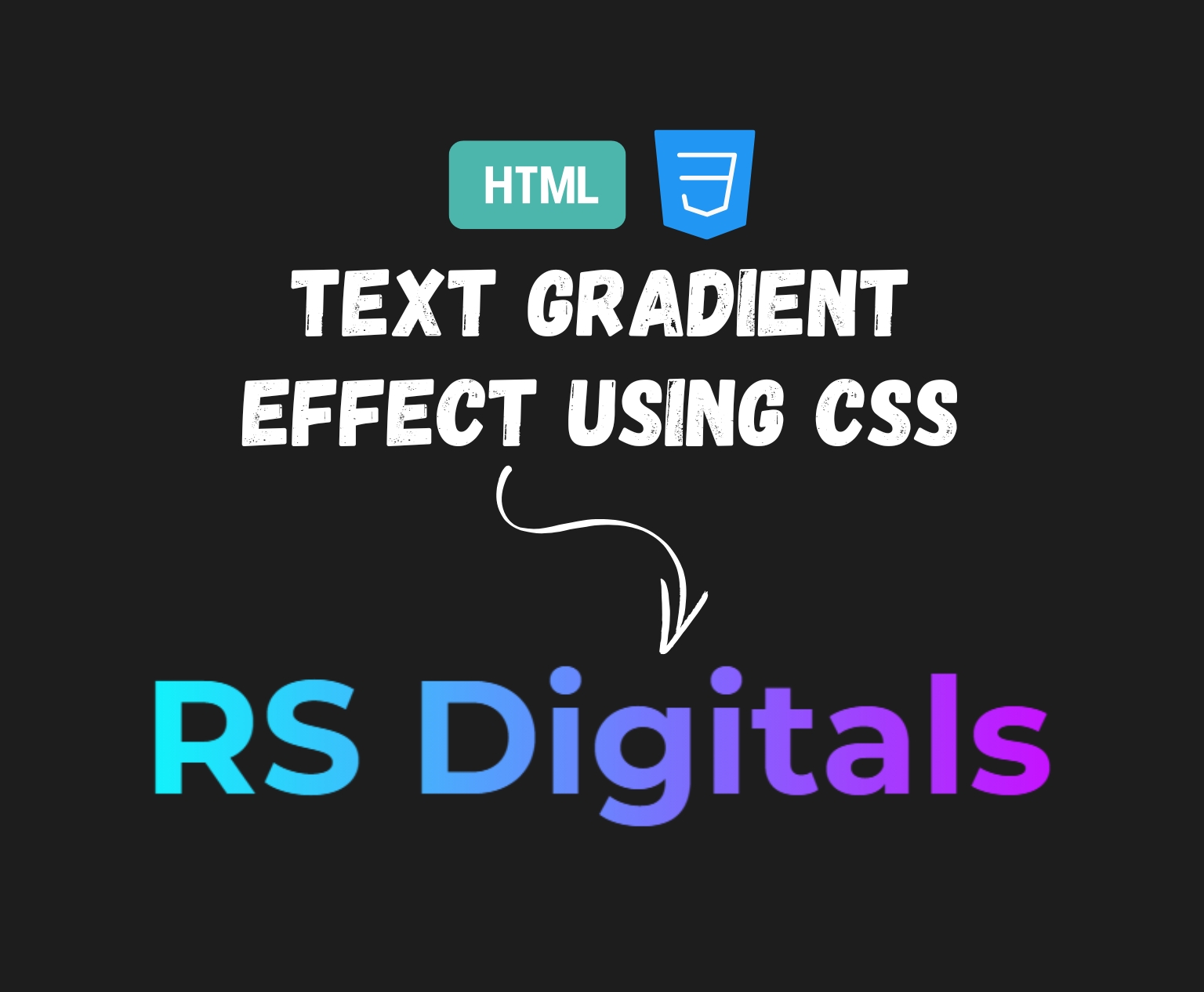
If you’ve ever scrolled through a website and been wowed by colorful, eye-catching text that seems to shift shades like a rainbow—chances are, you were looking at gradient text. It’s a simple yet powerful design technique that can instantly make your headlines or brand elements pop. In this post, I’ll walk you through how to create that same gradient text effect using just CSS—no Photoshop or fancy design software needed!
Setting up the HTML
Before we dive into the CSS, let’s take a quick look at the HTML structure. Here’s the complete code for setting up the page:
<!DOCTYPE html>
<html>
<head>
<title> CSS Gradient Effect </title>
<link href="style.css" rel="stylesheet" />
</head>
<body>
<h1 class="heading"> RS Digitals </h1>
</body>
</html>
Here’s a breakdown of what each part of the code does:
<!DOCTYPE html>
: This declaration tells the browser that we’re using HTML5 to structure the page.<html>
: This is the root element that wraps the entire HTML content.<head>
: Contains meta-information about the webpage, like the title and linked files.<title> CSS Gradient Effect </title>
: This sets the title of the webpage, which appears in the browser tab.<link href="style.css" rel="stylesheet" />
: This is linking to an external CSS file (style.css), where we’ll define the gradient styles.
<body>
: This section holds the visible content of the webpage.<h1 class="heading"> RS Digitals </h1>
: Here, we’re creating a header element with the text “RS Digitals” and applying the classheading
, which we’ll later target with CSS to add our gradient effect.
This is the basic HTML setup, and now that we have our structure, we’ll move on to the exciting part—applying the gradient effect with CSS!
CSS Code for the Gradient Text Effect:
body {
background-color: #1d1d1d;
}
.heading {
width: 100%;
margin: 150px 0px;
text-align: center;
font-size: 8vw;
font-family: "Montserrat";
background-image: linear-gradient(90deg, #08fffb 25%, #ce08ff 75%);
background-clip: text;
-webkit-background-clip: text;
-webkit-text-fill-color: transparent;
}
Here’s what each part of the CSS code does:
body { background-color: #1d1d1d; }
: This sets the background color of the entire page to a dark shade (#1d1d1d
) to make the text stand out more..heading { ... }
: This is the class where we apply our gradient effect. It targets theh1
element in the HTML (with the class “heading”).width: 100%;
: This ensures the element takes up the full width of its parent container (in this case, the body of the page).margin: 150px 0px;
: Adds space above and below the heading, pushing it away from the top and bottom edges.text-align: center;
: Centers the heading text horizontally on the page.font-size: 8vw;
: The8vw
unit means the font size is 8% of the viewport width, making it responsive. The text size will adjust based on how big the browser window is.font-family: "Montserrat";
: This applies the “Montserrat” font to the heading text.background-image: linear-gradient(90deg, #08fffb 25%, #ce08ff 75%);
: This is the key part that creates the gradient. Thelinear-gradient
function generates a gradient from left to right (90deg
), starting with a light cyan color (#08fffb
) and transitioning to a purple shade (#ce08ff
).background-clip: text;
: This ensures the gradient is clipped to the text, making it visible only within the letters.-webkit-background-clip: text;
: This is a vendor-specific prefix needed for Safari to support the background clipping effect.-webkit-text-fill-color: transparent;
: This makes the text itself transparent, so the gradient shows through and fills the text shape.
And there you have it! This CSS will apply a beautiful gradient effect to your text, giving it a modern and vibrant look.
See the Gradient Text Effect in Action
Want to see the gradient text effect come to life? Check out the live demo below! I’ve embedded a CodePen where you can see the effect in action. Play around with the code, tweak it, and see how changing colors or fonts can make the text look even cooler. It’s a great way to experiment and get a feel for how this technique works.
See the Pen Untitled by Rohail Ali (@Rohail1123) on CodePen.
Final Thoughts
Creating a gradient text effect with CSS is a fun and simple way to add some flair to your web design. It’s a technique that’s easy to implement and can make your text stand out, giving it a modern and colorful look. Whether you’re designing a brand new website or just adding a pop of color to your existing pages, this gradient effect can truly elevate the visual appeal of your text. So, try it out for yourself, customize it, and have fun experimenting with different color combinations!