CSS Trick: Grainy Text Effect Without JavaScript
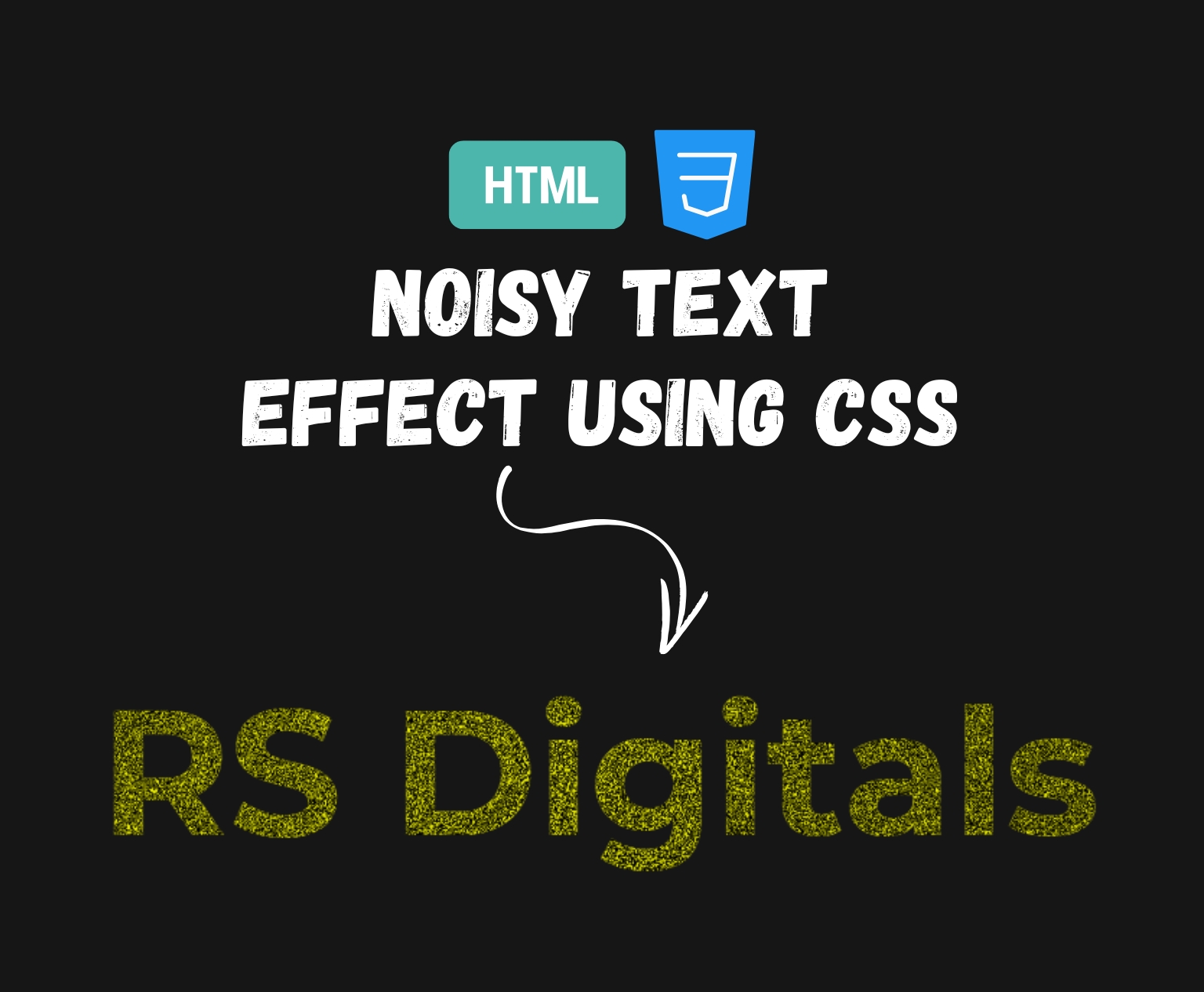
If you’re looking to add a subtle yet stylish texture to your website’s typography, a grainy text effect can be a great touch. And the best part? You don’t need any fancy graphic tools or external images—just good ol’ HTML and CSS. In this tutorial, I’ll walk you through how to create a grainy text effect using a simple combination of CSS filters, background layers, and blend modes. Whether you’re designing a landing page, blog header, or portfolio, this technique will give your text a unique, eye-catching flair. Let’s dive in!
HTML Code
<!DOCTYPE html>
<html>
<head>
<title>Text Grainy Effect</title>
<link href="style.css" rel="stylesheet">
</head>
<body>
<h1 class="grainy-text">RS Digitals</h1>
</body>
</html>
What’s Happening in This Code?
Let’s break it down quickly:
<!DOCTYPE html>
declares the document type and helps the browser render the page correctly.- The
<html>
tag wraps all the content on the page. - Inside the
<head>
, we have the<title>
tag which sets the title that appears on the browser tab (in this case, “Text Grainy Effect”). - The
<link>
tag connects an external CSS file (style.css
) where we’ll write the styles for our grainy effect. - Inside the
<body>
, we have a single<h1>
element with the classgrainy-text
. This is where our effect will be applied.
This HTML structure is minimal and clean—perfect for focusing solely on the visual effect we’re about to create. Next, we’ll move on to the CSS part, where all the magic happens.
CSS Code
body {
background-color: #161616;
}
.grainy-text {
font-size: 9vw;
font-weight: bold;
text-align: center;
font-family: "Montserrat";
color: transparent;
background:
repeating-radial-gradient(#161616 0 0.0001%, #fafd14 0 0.0002%) 30% 10% / 40vw 40vw,
repeating-conic-gradient(#161616 0 0.0001%, #fafd14 0 0.0002%) 70% 70% / 60vw 60vw;
background-clip: text;
-webkit-background-clip: text;
text-fill-color: transparent; /* For Safari */
background-blend-mode: difference;
margin: 120px 0;
}
What’s Happening in This CSS?
Let’s walk through the important parts:
body
background: We’re setting a dark background color (#161616
) for contrast so the grainy yellow pattern pops..grainy-text
styling:font-size: 9vw;
makes the text responsive, scaling with the width of the viewport.font-weight: bold;
andfont-family: "Montserrat";
give the text a modern, clean appearance.color: transparent;
makes the text itself invisible, allowing the background to show through.
- Background magic:
- We’re layering two repeating gradients:
repeating-radial-gradient
creates a speckled pattern using dark and bright colors in microscopic steps (0.0001% and 0.0002%).repeating-conic-gradient
adds additional variation and texture.
- These two backgrounds are positioned and sized differently to avoid looking too uniform, which adds to the natural, grainy look.
- We’re layering two repeating gradients:
- Text clipping:
background-clip: text;
and-webkit-background-clip: text;
ensure the gradient only fills the text, not the entire element box.text-fill-color: transparent;
is a Safari-specific rule to fully reveal the background inside the text.
background-blend-mode: difference;
:- This blend mode helps the two gradients interact in a way that increases the contrast and texture, giving it that “grain” effect.
- Margin: Adds vertical spacing to center the text on the page nicely.
This technique is great because it doesn’t rely on any external images or JavaScript. It’s lightweight, responsive, and works in modern browsers with good CSS support. Plus, it’s fully customizable—you can tweak the colors, gradient types, and blend modes to create your own unique variation of the effect.
See It Live
Want to see the grainy text effect in action? Check out the live demo below! I’ve embedded a CodePen preview so you can see exactly how the effect looks in the browser. Feel free to interact with the code, tweak the values, and experiment with your own custom styles. It’s a great way to learn by doing and get a feel for how these CSS properties work together to create a unique visual experience.
See the Pen Noisy Text Effect Using CSS by Rohail Ali (@Rohail1123) on CodePen.
Summary
In this tutorial, we explored how to create a stylish grainy text effect using just HTML and CSS—no JavaScript or images required. We started by setting up a simple HTML structure, then applied some powerful CSS techniques like repeating-gradient
, background-clip: text
, and blend-modes
to give our text a textured, eye-catching appearance.
This effect is lightweight, responsive, and fully customizable—perfect for adding creative flair to headers, banners, or hero sections on your website. Whether you’re a beginner experimenting with CSS or a seasoned developer looking to add some texture to your design, this trick is a great one to have in your toolkit.
Ready to make it your own? Scroll back up to the live demo and start playing with the code!